9. Hello World
Let's run through a basic example of controller and view interaction showing minimally necessary components. We will call a simple page that will say "Hello World" and will print out any name we pass via a 'name' query string parameter.
Let's first use the Basic Template for this example.
Here is the complete URL as viewed in my browser:
http://yii-basic.loc/hello-world/say-hi?name=JB
In this example, we are calling a controller named HelloWorldController.php and executing the action SayHi. We are also passing a name parameter that will be echoed out on the view page.
Notice the use of dashes in the url to specify the HelloWorld controller and the SayHi action. This is to match the camel case.
9.1 The Controller File
The following code will be saved as file /controllers/HelloWorldController.php
<?php namespace app\controllers; use Yii; use yii\web\Controller; class HelloWorldController extends Controller { public function actionSayHi($name='') { return $this->render('hello',[ 'name' => $name ]); } }
Items to note:
First, notice the namespace on line 3. This is essential.
Next, notice the use statements on lines 5 and 6. Since we are extending the Controller class, we must specify using that class.
You could actually forgone the use statements and changed line 8 as follows:
class HelloWorldController extends \yii\web\Controller
However, it is much more convenient to employ the use statements. But, you will see it both ways in your Yii travels.
On line 10, we are defining the SayHi action as a public function. The parameter $name is being passed to this function. This will access any query string parameter with 'name' as the key.
In line 13 we are rendering the hello view (which accesses the file hello.php in the directory 'hello-world' and are passing the $name parameter to the view.
As we have not specified an alternate template, the /views/layouts/main.php will be used. If we wanted to specify another template to use, we can do so. Let's say the alternate template you want to use is called front.php. This template will go in the same directory as main.php. You would specify the action to use this template by adding the following line to the actionSayHi function:
$this->layout = 'front';
9.2 The View File
Next, we need to create the view folder. Add the following directory to your application:
/basic/views/hello
Save the following code as hello.php in the hello-world directory:
<?php use yii\helpers\Html; $this->title = 'Hello World'; $this->params['breadcrumbs'][] = $this->title; ?> <div> <h1><?= Html::encode($this->title) ?></h1> <p> Testing Yii. My name is: <?=$name?> </p> </div>
Items to note:
On line 9, we are using a HTML helper function. This is to HTML encode the value passed to it. To use this, we are importing the Html helper class on line 3.
We are setting the page title on line 5, which will be echoed out in using the h1 tags on line 9 and also be used as the page title attribute.
On line 12, we are echoing out the $name variable. This variable was passed to us through the controller, as discussed.
9.3 The Output
Here is a screen shot of how the code above is rendered:
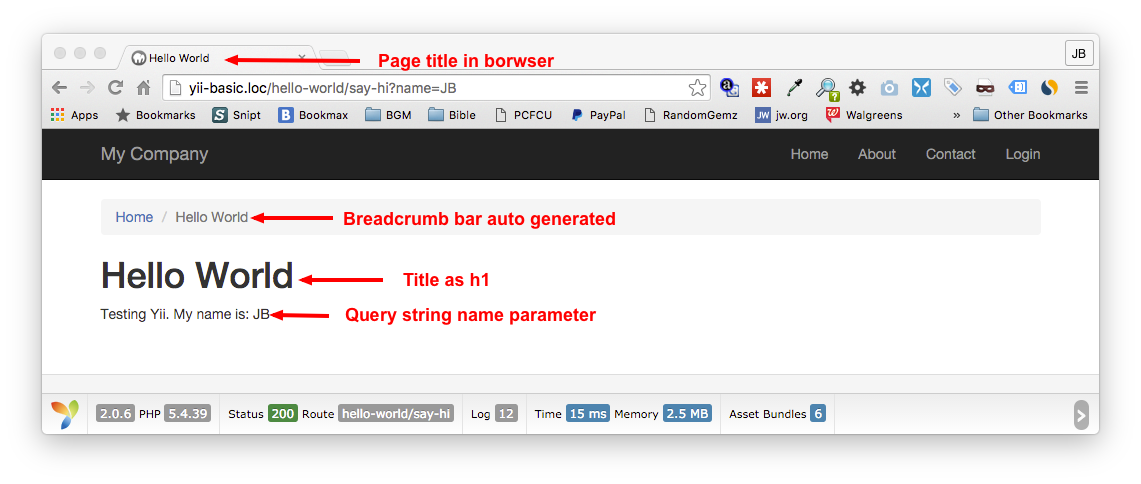
9.4 Hello World Using Advanced Template
If we are using the Advanced Template, you will modify the above as follows:
Let's put our Hello World example in the frontend section. Copy HelloWorldController.php and put it in the /frontend/controllers directory. Copy the hello-world directory and put it in /frontend/views directory.
You will end up with the following paths:
Controller: /frontend/controllers/HelloWorldController.php
View: /frontend/views/hello-world/hello.php
Let's execute this code in our browser and see what we get. In my setup, the URL is: http://yii-advanced-frontend.loc/hello-world/say-hi?name=JB
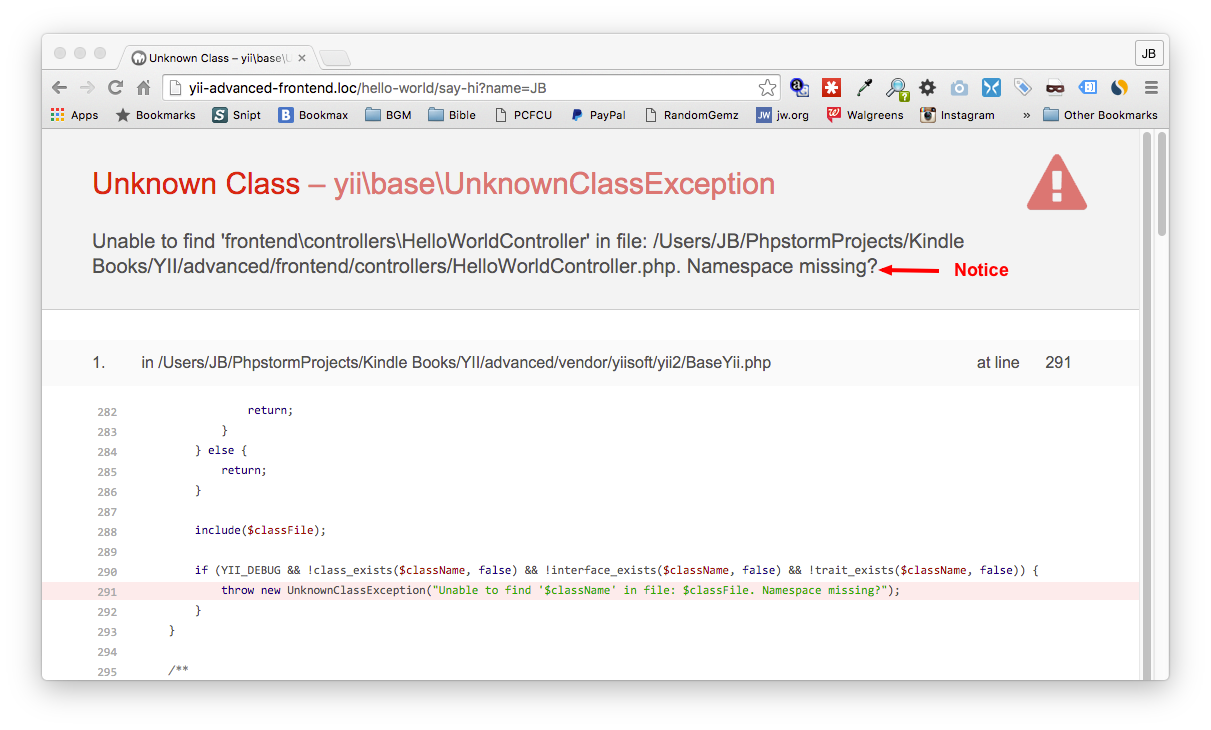
Uh oh. We got an error. What went wrong?
Remember when we created the controller for the basic template? We have the following on line 3: namespace app\controllers;
The issue is, since we are no longer in the basic template, the name space is wrong. The error message signifies this fact. We need to change line 3 as follows:
namespace frontend\controllers;